In the world of Unix-like operating systems, two prominent shells stand out: /bin/sh and /bin/bash. These seemingly similar terms often lead to confusion among newcomers and even seasoned users. In this comprehensive guide, we’ll explore the critical distinctions between these two shells, their functionality, use cases, and why understanding them is essential for any Linux enthusiast.
Unraveling the Shell Universe
Shell Basics
To begin our exploration, let’s start with the fundamentals. Both /bin/sh and /bin/bash serve as command-line interpreters or shells. They facilitate interaction between users and the operating system, allowing users to execute commands, run scripts, and manipulate system resources.
The Classic /bin/sh
Origin and Simplicity
/bin/sh, short for Bourne Shell, was one of the earliest Unix shells developed by Stephen Bourne in the 1970s. It embodies simplicity and efficiency, making it a lightweight choice for system processes and scripts.
Key Characteristics
- Minimalistic: /bin/sh is minimalistic, providing only essential functionality;
- Portability: It’s available on nearly all Unix-like systems;
- Scripting: Primarily used for scripting system tasks;
- Speed: Offers quick execution, ideal for resource-constrained environments.
The Versatile /bin/bash
A Bashful Beginning
On the other hand, /bin/bash, or the Bourne-Again Shell, emerged as an enhanced version of /bin/sh. Brian Fox developed it in the late 1980s, combining features from various Unix shells.
Key Features
- Extensibility: /bin/bash is highly extensible, supporting customizations and user-defined functions;
- Interactive Use: It provides an excellent interactive command-line experience;
- Scripting Powerhouse: Widely used for scripting and automation, offering a plethora of built-in commands and features;
- Compatibility: It retains compatibility with /bin/sh, making it a suitable upgrade.
Choosing the Right Shell
When deciding between /bin/sh and /bin/bash, it’s crucial to consider your specific use case.
Use Cases
- System Boot Scripts: /bin/sh is often preferred for boot scripts to ensure minimal overhead;
- Interactive Sessions: For day-to-day interactive use, /bin/bash’s enhanced features shine;
- Scripting Complexity: When your scripts require advanced functionality, /bin/bash is the go-to choice;
- Cross-Platform Scripts: If cross-compatibility is essential, /bin/sh is a safer bet.
Compatibility and Legacy Concerns
Keep in mind that while /bin/bash is more versatile, it may not be available on all systems. If compatibility with a wide range of Unix-like systems is crucial, it’s wise to stick with /bin/sh.
Key Differences at a Glance
Let’s summarize the critical differences between /bin/sh and /bin/bash in a convenient table:
Aspect | /bin/sh | /bin/bash |
---|---|---|
Origin | Bourne Shell | Bourne-Again Shell |
Scripting Power | Basic scripting capabilities | Advanced scripting and automation features |
Interactive Use | Limited interactivity | Rich interactive command-line experience |
Compatibility | Highly portable | May require installation on some systems |
Extensibility | Minimal extensibility | Highly extensible and customizable |
Code Snippet
Here’s a simple code snippet illustrating the difference in echo behavior between /bin/sh and /bin/bash:
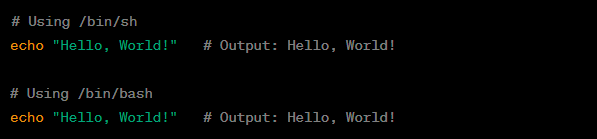
Digging Deeper into /bin/sh
Under the Hood
To truly appreciate the role of /bin/sh in Unix systems, it’s beneficial to delve into its inner workings. This shell, often referred to as the “Bourne Shell” after its creator Stephen Bourne, is designed to be minimalistic. Its primary goal is to execute commands efficiently while conserving system resources. This efficiency makes it an ideal choice for system initialization scripts, where speed and simplicity are paramount.
Legacy and Portability
One of the standout features of /bin/sh is its exceptional portability. Thanks to its minimalist design, it can be found on virtually every Unix-like system, ensuring that scripts written for /bin/sh will run consistently across various platforms. This legacy compatibility has cemented its status as a foundational shell in the Unix world.
Use Cases Revisited
While /bin/sh excels in simplicity and portability, its limitations become apparent when faced with more complex scripting requirements. Its lack of advanced features like arrays and enhanced flow control mechanisms can hinder script development. Therefore, it’s crucial to consider the specific demands of your task when choosing between /bin/sh and /bin/bash.
Unleashing the Power of /bin/bash
The Bash Advantage
/bin/bash, often known as the “Bourne-Again Shell,” builds upon the foundation of /bin/sh with a wealth of additional features and capabilities. This enhanced shell is a powerhouse for interactive command-line use and scripting. It provides an extensive set of built-in commands and supports user-defined functions, making it the preferred choice for complex tasks.
Scripting Mastery
One of the key strengths of /bin/bash lies in its scripting capabilities. Scriptwriters can take advantage of advanced features like arrays, associative arrays, and powerful flow control constructs, allowing for the creation of robust and feature-rich scripts. This scripting prowess makes /bin/bash indispensable for automation tasks and application scripting.
Interactive Bliss
For those who spend significant time on the command line, the interactive experience offered by /bin/bash is unparalleled. It boasts features like tab completion, command history, and customizable prompt strings, enhancing efficiency and convenience. This makes it the go-to choice for system administrators, developers, and power users.
Making the Choice
Decision Factors
As you weigh the pros and cons of /bin/sh and /bin/bash, consider your specific needs and constraints. If you’re developing system initialization scripts or require maximum portability, /bin/sh is a pragmatic choice. On the other hand, if your tasks involve intricate scripting, interactive use, or automation, /bin/bash offers the versatility and power required.
Combining the Best of Both Worlds
In some scenarios, you may find it advantageous to use both shells within your Unix environment. For example, you can write your system startup scripts in /bin/sh for efficiency and compatibility while leveraging /bin/bash for user-specific customization and automation.
Exploring Alternatives
While /bin/sh and /bin/bash are two of the most widely used Unix shells, there are alternative shells worth exploring. Shells like /bin/zsh, known for its advanced features and scripting capabilities, and /bin/fish, appreciated for its user-friendliness, cater to specific needs and preferences. Experimenting with these alternatives can offer fresh insights into shell usage.
With a deeper understanding of /bin/sh and /bin/bash, you’re better equipped to navigate the Unix shell landscape and make informed choices that align with your computing objectives. Whether you embrace the simplicity of /bin/sh or harness the power of /bin/bash, your proficiency in Unix-like systems will undoubtedly benefit from this knowledge.
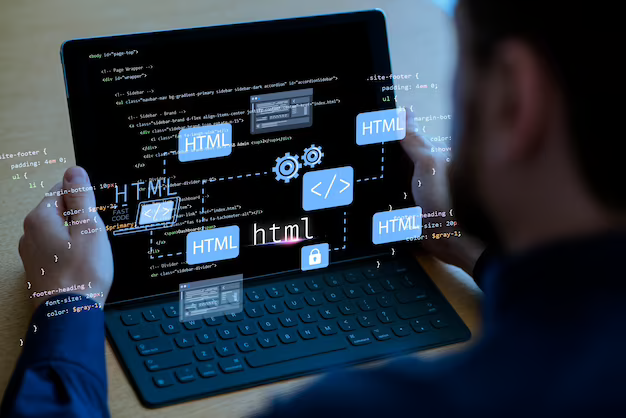
Environmental Variables
Understanding Variables
In the context of shells like /bin/sh and /bin/bash, environmental variables play a crucial role in defining system behavior and customizing user experiences. These variables are essentially placeholders that hold information such as system paths, user preferences, and configuration settings.
Common Environmental Variables
Here are some frequently used environmental variables and their roles:
- PATH: This variable lists directories where the shell looks for executable files. It determines which commands can be run without specifying their full path.
- HOME: It points to the current user’s home directory, making it easy to reference personal files and settings.
- USER: Identifies the current user’s username, useful for scripts and configuration.
- SHELL: Specifies the default shell for the user, helping to ensure a consistent shell environment.
Customizing Variables
Users can modify and create their environmental variables to suit their needs. This level of customization allows for tailoring the shell experience, setting default values for specific applications, or defining variables needed for custom scripts.
Scripting Examples
Basic Scripting
Let’s delve into some practical examples to illustrate the difference in scripting between /bin/sh and /bin/bash.
Example 1: Hello World
Here’s a simple “Hello World” script in /bin/sh:

And the same script in /bin/bash:

Both scripts accomplish the same task, but /bin/bash allows for more advanced functionality as scripts become more complex.
Example 2: Variables
In /bin/sh, setting and using variables is straightforward:

However, in /bin/bash, you have additional features like arrays and more robust variable manipulation:
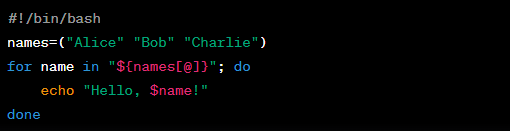
Advanced Scripting
For advanced scripting needs, /bin/bash offers a wider array of tools, such as regular expressions, arithmetic operations, and complex data structures. These capabilities can significantly simplify complex scripting tasks.
Security Considerations
Shell Security
While both /bin/sh and /bin/bash are powerful tools, they can also pose security risks when misused. It’s essential to follow best practices to minimize vulnerabilities:
1. Limit Permissions
Ensure that scripts and shell configurations have the appropriate permissions to prevent unauthorized access or modification.
2. Validate User Input
Sanitize user input to prevent command injection attacks. Always validate and sanitize data before using it in shell commands.
3. Use Safe Practices
Avoid running scripts with elevated privileges unless necessary. Employ least privilege principles to minimize potential damage.
4. Keep Software Updated
Regularly update your shell and system software to patch security vulnerabilities.
5. Audit Scripts
Regularly audit and review scripts for potential security issues. Use tools like ShellCheck to identify and fix problems.
Conclusion
In the realm of Unix-like operating systems, the choice between /bin/sh and /bin/bash is pivotal. Understanding their differences empowers you to make informed decisions, ensuring optimal performance and compatibility for your tasks. Whether you opt for the simplicity of /bin/sh or the versatility of /bin/bash, both shells play vital roles in the Unix ecosystem.
Please support us by hitting the like button on this prompt. This will encourage us to further improve this prompt to give you the best results.
Frequently Asked QuestionsÂ
No, /bin/bash may not be available on all Unix-like systems by default. You might need to install it separately.
Yes, you can generally use /bin/bash syntax in /bin/sh scripts, as /bin/bash is designed to be compatible with /bin/sh.
For system maintenance scripts where minimalism and speed are essential, /bin/sh is often preferred.
Yes, there are several alternative shells, such as /bin/zsh and /bin/fish, each with its own unique features and characteristics.
The default shell in many Unix-like systems is /bin/bash, but this can vary depending on the distribution and system configuration.